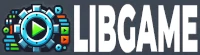 |
LibGame
v0.4.0
The LG Game Engine - Copyright (C) 2024 ETMSoftware
|
23 #define TIME_FILE_LINE_FUNC_STR "%lld [%s: %d] %s(): ", lg_log_time(), __FILE__ , __LINE__, __func__
25 #define INFO_ERR2(...) \
27 fprintf(STD_ERR, TIME_FILE_LINE_FUNC_STR);\
28 fprintf(STD_ERR, __VA_ARGS__);\
33 #define LG_ERR_CTX_FILE_MAXLEN 63
34 #define LG_ERR_CTX_FUNC_MAXLEN 63
35 #define LG_ERR_CTX_TXT_MAXLEN 1023
36 #define LG_ERR_CTX_CODE_NA (-100000)
37 #define LG_ERR_CTX_STR_MAXLEN 2048
38 #define LG_ERR_CTX_FORMAT "[%s: %d] %s(): %s (code = %d)"
41 char file[LG_ERR_CTX_FILE_MAXLEN + 1];
43 char func[LG_ERR_CTX_FUNC_MAXLEN + 1];
44 char txt[LG_ERR_CTX_TXT_MAXLEN + 1];
48 enum {OOM_MALLOC2_TEST, OOM_MALLOC3_TEST, INVPTR_FREE2_TEST, INVPTR_FREE3_TEST, SEGFAULT_TEST, DIVBYZERO_TEST};
65 void *
malloc2_plus(
size_t,
const char *,
unsigned int,
const char *);
66 #define malloc3(...) malloc2_plus(__VA_ARGS__, __FILE__, __LINE__, __func__)
68 void free2_plus(
void *,
const char *,
unsigned int,
const char *);
72 #define free2_ultimate(...) free2_plus(__VA_ARGS__, __FILE__, __LINE__, __func__)
73 #define free3(mem_block) {free2_ultimate(mem_block); mem_block = NULL;}
79 int mkdir_plus(
const char *, mode_t,
const char *,
unsigned int,
const char *);
81 #define mkdir_2(...) mkdir(__VA_ARGS__)
84 FILE *
fopen_plus(
const char *,
const char *,
const char *,
unsigned int,
const char *);
86 #define fopen_2(...) fopen(__VA_ARGS__)
void test_big_error(int test)
Definition: lg_error.c:388
void lg_set_error_context(const char *file, unsigned int line, const char *func, const char *txt, int code)
Definition: lg_error.c:128
int mkdir_plus(const char *path, mode_t mode, const char *file, unsigned int line, const char *func)
Definition: lg_error.c:263
void set_sig_handler()
Definition: lg_error.c:362
void lg_save_error_context(LG_ErrorContext *err_ctx)
Definition: lg_error.c:181
long long lg_log_time()
Definition: lg_error.c:116
void sig_handler(int sig_num, siginfo_t *sig_info, void *context)
Definition: lg_error.c:306
const char * lg_get_full_error_context()
Definition: lg_error.c:152
void * malloc2_plus(size_t size, const char *file, unsigned int line, const char *func)
Definition: lg_error.c:230
void lg_clear_error_context()
Definition: lg_error.c:165
Definition: lg_error.h:40
void free2_plus(void *mem, const char *file, unsigned int line, const char *func)
Definition: lg_error.c:247
int lg_get_error_context_code()
Definition: lg_error.c:142
void lg_restore_error_context(LG_ErrorContext *err_ctx)
Definition: lg_error.c:189
FILE * fopen_plus(const char *path, const char *mode, const char *file, unsigned int line, const char *func)
Definition: lg_error.c:282