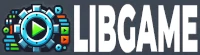 |
LibGame
v0.4.0
The LG Game Engine - Copyright (C) 2024-2025 ETMSoftware
|
109 uint32_t lg_point2df_is_inside_rec2df_from_array(
Point2Df,
Rec2Df **, uint32_t);
int32_t sdl_to_gl_int_x(int32_t x)
Definition: lg_gr_func.c:548
Point2Df lg_point2d_f(float x, float y)
Definition: lg_gr_func.c:364
Point2Df lg_rec_f_center(Rec2Df r)
Definition: lg_gr_func.c:388
Definition: lg_gr_func.h:56
Definition: lg_gr_func.h:20
void logical_coords_from_touchscreen_coords(int *x2, int *y2, float x1, float y1)
Definition: lg_gr_func.c:467
int32_t sdl_to_gl_int_y(int32_t y)
Definition: lg_gr_func.c:559
void lg_rec_f_copy(Rec2Df *dest, Rec2Df src)
Definition: lg_gr_func.c:264
LG_Color_u lg_get_lg_color_u_from_str(const char *str)
Definition: lg_gr_func.c:686
SDL_Color lg_sdl_color_from_lg_color_u(LG_Color_u c)
Definition: lg_gr_func.c:635
Definition: lg_gr_func.h:15
Point2Di lg_point2d_i(int32_t x, int32_t y)
Definition: lg_gr_func.c:352
SDL_Color lg_get_sdl_color_from_str(const char *str)
Definition: lg_gr_func.c:666
Definition: lg_gr_func.h:32
zboolean lg_recs_overlap(Rec2Df *r1, Rec2Df *r2)
Definition: lg_gr_func.c:219
const char * lg_get_color_str_from_color_index(int i)
Definition: lg_gr_func.c:706
void lg_clear_rect(Rec2Di r, LG_Color_u c)
Definition: lg_gr_func.c:116
void lg_draw_rect(Rec2Di r, LG_Color_u c)
Definition: lg_gr_func.c:46
void lg_set_touchscreen_coords_margins(float left, float top)
Definition: lg_gr_func.c:488
Rec2Di lg_rec_i_from_rec_f(Rec2Df r)
Definition: lg_gr_func.c:333
void logical_coords_from_viewport_coords(int *x2, int *y2, int x1, int y1)
Definition: lg_gr_func.c:428
LG_Color_u * lg_set_lg_color_u_from_str(LG_Color_u *c, const char *str)
Definition: lg_gr_func.c:677
Rec2Di lg_rec_i(int x, int y, int w, int h)
Definition: lg_gr_func.c:281
LG_Color_u lg_color_u_from_sdl_color(SDL_Color c)
Definition: lg_gr_func.c:624
void lg_fill_rect(Rec2Di r, LG_Color_u c)
Definition: lg_gr_func.c:89
Point2Di lg_rec_i_center(Rec2Di r)
Definition: lg_gr_func.c:377
void lg_draw_line(int32_t x1, int32_t y1, int32_t x2, int32_t y2, LG_Color_u c)
Definition: lg_gr_func.c:30
Rec2Df lg_rec_f(float x, float y, float w, float h)
Definition: lg_gr_func.c:302
Definition: lg_vertex.h:111
Definition: lg_gr_func.h:25
float sdl_to_gl_float_y(int32_t y)
Definition: lg_gr_func.c:588
float sdl_to_gl_float_x(int32_t x)
Definition: lg_gr_func.c:574
void v_flip_sdl_surf(SDL_Surface *surf)
Definition: lg_gr_func.c:729
void lg_copy_rect(Rec2Di src, Rec2Di *dest)
Definition: lg_gr_func.c:236
void logical_coords_from_viewport_coords_btaskbar_adjustment(int bth_adj)
Definition: lg_gr_func.c:446
Rec2Df lg_rec_f_from_rec_i(Rec2Di r)
Definition: lg_gr_func.c:320
zboolean lg_clip_rect(Rec2Di clip, Rec2Di rect, Rec2Di *clipped_rect)
Definition: lg_gr_func.c:163
void lg_rec_i_copy(Rec2Di *dest, Rec2Di src)
Definition: lg_gr_func.c:250
void gl_coords_from_screen_coords(float *x2, float *y2, int x1, int y1, int viewport_w, int viewport_h)
Definition: lg_gr_func.c:406
SDL_Color * lg_set_sdl_color_from_str(SDL_Color *c, const char *str)
Definition: lg_gr_func.c:643
uint32_t lg_point2di_is_inside_rec2di_from_array(Point2Di p, Rec2Di **r, uint32_t n_rect_max)
Definition: lg_gr_func.c:504
Definition: lg_gr_func.h:49
int lg_get_n_color_str()
Definition: lg_gr_func.c:695