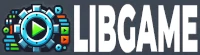 |
LibGame
v0.4.0
The LG Game Engine - Copyright (C) 2024-2025 ETMSoftware
|
10 # define FLUSH_KEYB_OR_TOUCHSCREEN_BUF {lg_flush_keyb_events();}
12 # define FLUSH_KEYB_OR_TOUCHSCREEN_BUF {lg_flush_touch_events();}
16 # define WAIT_FOR_PRESS_OR_TOUCH {lg_wait_for_any_key_pressed();}
18 # define WAIT_FOR_PRESS_OR_TOUCH {lg_wait_for_finger_down(NULL, NULL);}
21 #define SPECIAL_KEYS_REPEAT_DELAY 200
24 #define LPK_MOUSE_CLICK_ACTIVITY 50000
26 #define LPK_TOUCH_ACTIVITY 100000
30 uint8_t arrow_keys_state;
34 uint32_t last_pressed_key;
36 lg_finger_down_loc finger_down_loc;
83 #define TZW_ARROW_POINTS_NUM 7
92 TZW_DOUBLE_ARROW_HORIZ,
93 TZW_DOUBLE_ARROW_VERT,
100 #define N_TZW_MAX 256
void lg_draw_arrow(Rec2Di r, LG_Color_u c, lg_tzw_type type)
Definition: lg_ui.c:549
const char ** lg_camcontrols_keys_str()
Definition: lg_ui.c:108
Rec2Di lg_tzwin_get_area(LG_TouchZoneWindow *tzwin)
Definition: lg_ui.c:501
void lg_tzwin_show(LG_TouchZoneWindow *tzwin, lg_tzw_type type)
Definition: lg_ui.c:434
void lg_rectangle_to_arrow(Rec2Di r, lg_tzw_type arrow_type, Point2Di *p[])
Definition: lg_ui.c:572
void lg_draw_rect_corners(Rec2Di r, LG_Color_u c, int len)
Definition: lg_ui.c:518
void lg_set_special_keys_repeat_delay(uint32_t delay)
Definition: lg_ui.c:729
Definition: lg_gr_func.h:15
LG_TZW_Array * lg_tzw_array_new()
Definition: lg_ui.c:786
void lg_flush_in_clicks(LG_InputState *in)
Definition: lg_ui.c:887
void lg_tzw_array_free(LG_TZW_Array *tzw_array)
Definition: lg_ui.c:877
void lg_draw_triangle(Rec2Di r, LG_Color_u c, lg_tzw_type type)
Definition: lg_ui.c:695
zboolean lg_tzwin_add_to_array(LG_TZW_Array *tzw_array, LG_TouchZoneWindow *tzwin)
Definition: lg_ui.c:805
Definition: lg_vertex.h:111
void lg_tzw_array_free_all_tzwin_after(LG_TZW_Array *tzw_array, uint32_t next_index)
Definition: lg_ui.c:858
void lg_input_state_info(LG_InputState *in)
Definition: lg_ui.c:900
LG_TouchZoneWindow * lg_tzwin_new(const char *str, zboolean no_margin, LG_Color_u text_color, int x_loc, int y_loc, TTF_Font *f)
Definition: lg_ui.c:359
LG_TZRec_Array lg_rec2di_array_from_tzw_array(LG_TZW_Array *tzw_array)
Definition: lg_ui.c:825
void lg_tzw_array_free_all_tzwin(LG_TZW_Array *tzw_array)
Definition: lg_ui.c:847
uint32_t lg_get_user_input(LG_InputState *in, LG_TZW_Array *tzw_array)
Definition: lg_ui.c:179
void lg_tzwin_free(LG_TouchZoneWindow *tzwin)
Definition: lg_ui.c:488
Definition: lg_gr_func.h:49