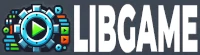 |
LibGame
v0.4.0
The LG Game Engine - Copyright (C) 2024-2025 ETMSoftware
|
9 #define LG_MESH_MAX_WEIGHTS 4
16 typedef struct __attribute__((packed, aligned(4))) {
23 typedef struct __attribute__((packed, aligned(4))) {
32 typedef struct __attribute__((packed, aligned(4))) {
46 typedef struct __attribute__((packed, aligned(4))) {
62 typedef struct __attribute__((packed, aligned(4))) {
72 uint8_t i[LG_MESH_MAX_WEIGHTS];
73 uint16_t w[LG_MESH_MAX_WEIGHTS];
80 typedef struct __attribute__((packed, aligned(4))) {
96 typedef struct __attribute__((packed, aligned(4))) {
111 typedef struct __attribute__((packed, aligned(4))) {
119 typedef struct __attribute__((packed, aligned(4))) {
LG_Color_u lg_color_u_from_f(LG_Color_f c)
Definition: lg_vertex.c:242
Definition: lg_vertex.h:23
Vertex_uv lg_vertex_uv(float x, float y, float z, uint16_t u, uint16_t v)
Definition: lg_vertex.c:75
Definition: lg_vertex.h:96
Vertex lg_vertex_from_vec3(vec3_t v)
Definition: lg_vertex.c:54
Definition: lg_vertex.h:46
Vertex_n lg_vertex_n(float x, float y, float z, int16_t n_x, int16_t n_y, int16_t n_z)
Definition: lg_vertex.c:93
Definition: lg_vertex.h:32
LG_Color_f lg_color_f(float r, float g, float b, float a)
Definition: lg_vertex.c:224
Definition: lg_vertex.h:16
Definition: math_3d.h:123
Definition: lg_vertex.h:119
void lg_vertex_sizes_info()
Definition: lg_vertex.c:268
Definition: lg_vertex.h:111
Vertex_rgba lg_vertex_rgba(float x, float y, float z, LG_Color_u c)
Definition: lg_vertex.c:159
Vertex_rgba_n lg_vertex_rgba_n(float x, float y, float z, LG_Color_u c, int16_t n_x, int16_t n_y, int16_t n_z)
Definition: lg_vertex.c:192
Vertex lg_vertex(float x, float y, float z)
Definition: lg_vertex.c:37
Vertex_rgba lg_vertex_rgba_from_vec3(vec3_t v, LG_Color_u c)
Definition: lg_vertex.c:173
Definition: lg_vertex.h:62
Definition: lg_vertex.h:80
Vertex_uvn_iw lg_vertex_uvn_iw(float x, float y, float z, uint16_t u, uint16_t v, int16_t n_x, int16_t n_y, int16_t n_z, uint8_t i[4], uint16_t w[4])
Definition: lg_vertex.c:141
LG_Color_u lg_color_u(uint8_t r, uint8_t g, uint8_t b, uint8_t a)
Definition: lg_vertex.c:208
Vertex_uv_n lg_vertex_uv_n(float x, float y, float z, uint16_t u, uint16_t v, int16_t n_x, int16_t n_y, int16_t n_z)
Definition: lg_vertex.c:115
LG_Color_f lg_color_f_from_u(LG_Color_u c)
Definition: lg_vertex.c:258