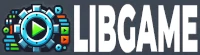 |
LibGame
v0.4.0
The LG Game Engine - Copyright (C) 2024-2025 ETMSoftware
|
33 #define LG_AUDIO_FREQ 48000
34 #define LG_AUDIO_FORMAT AUDIO_S16SYS
35 #define LG_AUDIO_N_CHANNELS 2
36 #define LG_AUDIO_BUF_SIZE (2 * 1024)
38 #define LG_AUDIO_MAX_VOL MIX_MAX_VOLUME
40 #define SOUND_FILENAME_MAXLEN (64 - 1)
43 char file_name[SOUND_FILENAME_MAXLEN + 1];
49 LG_PLAY, LG_REPEAT, LG_WAIT, LG_STOP, LG_PAUSE, LG_RESUME
int lg_load_play_music(const char *file_name, int loops)
Definition: lg_audio.c:72
void lg_audio_pause()
Definition: lg_audio.c:393
float lg_sound_channel_get_volume(LG_Sound *sound)
Definition: lg_audio.c:313
LG_Sound * lg_sound_new(const char *file_name)
Definition: lg_audio.c:145
int lg_audio_get_n_channels()
Definition: lg_audio.c:419
void lg_enable_cant_play_audio_file()
Definition: lg_audio.c:179
Definition: lg_audio.h:42
void lg_resume_music()
Definition: lg_audio.c:108
void lg_disable_cant_play_audio_file()
Definition: lg_audio.c:169
int lg_init_audio_to_defaults()
Definition: lg_audio.c:25
zboolean lg_music_is_playing()
Definition: lg_audio.c:90
void lg_sound_free(LG_Sound *sound)
Definition: lg_audio.c:327
void lg_pause_music()
Definition: lg_audio.c:98
int lg_init_audio(int freq, uint16_t format, int n_channels, int buffer_size)
Definition: lg_audio.c:45
void lg_free_audio()
Definition: lg_audio.c:60
void lg_free_music()
Definition: lg_audio.c:130
void lg_audio_resume()
Definition: lg_audio.c:401
void lg_stop_music()
Definition: lg_audio.c:118
zboolean lg_sound_is_playing(LG_Sound *sound)
Definition: lg_audio.c:279
int lg_sound_play(lg_play_mode mode, LG_Sound *sound)
Definition: lg_audio.c:233
void lg_sound_channel_set_volume(LG_Sound *sound, float v)
Definition: lg_audio.c:296
void lg_audio_set_sounds_volume(float v)
Definition: lg_audio.c:378
void lg_audio_set_music_volume(float v)
Definition: lg_audio.c:361
void lg_audio_set_global_volume(float v)
Definition: lg_audio.c:344