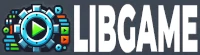 |
LibGame
v0.4.0
The LG Game Engine - Copyright (C) 2024 ETMSoftware
|
18 #ifndef _ISOC11_SOURCE
19 #define _ISOC11_SOURCE
22 #define LIBGAME_NAME "LibGame"
23 #define LIBGAME_V_NUM "0.4.0"
24 #define LIBGAME_COPYRIGHT_STR "Copyright (C) Emmanuel Thomas-Maurin 2012-2024 - All rights reserved"
26 #define LIBGAME_WEBSITE "https://www.etmsoftware.com"
27 #define LIBGAME_DOWNLOAD_WEBSITE LIBGAME_WEBSITE "/download.php"
28 #define LIBGAME_SUPPORT_WEBSITE LIBGAME_WEBSITE "/help.php"
31 #if !defined(ANDROID_V) && !defined(WIN32_V)
57 #include <android/log.h>
62 #include <SDL_image.h>
63 #include <SDL_mixer.h>
65 #include <SDL_syswm.h>
67 #include <SDL_mouse.h>
71 #include <SDL2/SDL_image.h>
72 #include <SDL2/SDL_mixer.h>
73 #include <SDL2/SDL_ttf.h>
74 #include <SDL_mouse.h>
75 #include <SDL_syswm.h>
78 #include <GLES2/gl2.h>
79 #include <GLES2/gl2ext.h>
82 #define LIBETM_VERBOSE_OUTPUT
86 #ifdef LIBETM_DEBUG_OUTPUT
87 #ifndef LIBETM_VERBOSE_OUTPUT
88 #define LIBETM_VERBOSE_OUTPUT
104 #include "../extra_libs/libetm-0.5.1/libetm.h"
107 #include <libetm/libetm.h>
109 #include "../extra_libs/libetm-0.5.1/libetm.h"
115 #define INFO_ERR INFO_ERR2
119 #define VERBOSE_OUTPUT
124 #ifndef VERBOSE_OUTPUT
125 #define VERBOSE_OUTPUT
131 #define TMPSTR_SIZE ((4 * 1024) - 1)
132 #define FILE_NAME_MAXLEN TMPSTR_SIZE
134 #ifdef LIBGAME_COMPILE_TIME
135 #include "../extra_libs/math_3d.h"
136 #include "../extra_libs/stb_image.h"
138 #include <libgame/extra_libs/math_3d.h>
139 #include <libgame/extra_libs/stb_image.h>
145 #include "lg_shader_progs.h"
146 #include "lg_vertex.h"
147 #include "lg_gr_func.h"
148 #include "lg_textures.h"
149 #include "lg_dds_loader.h"
150 #include "lg_astc_loader.h"
151 #include "lg_linked_list.h"
152 #include "lg_sprites.h"
153 #include "lg_background.h"
155 #include "lg_keyboard.h"
156 #include "lg_mouse.h"
157 #include "lg_touchscreen.h"
158 #include "lg_audio.h"
159 #include "lg_dirs_stuff.h"
160 #include "lg_quaternions.h"
163 #include "lg_3d_primitives.h"
164 #include "lg_opengl_2d.h"
166 #include "lg_camera.h"
167 #include "lg_obj_parser.h"
168 #include "lg_light.h"
169 #include "lg_scene_graph.h"
170 #include "lg_scene.h"
171 #include "lg_collision_detect.h"
172 #include "lg_admob.h"
173 #include "lg_vao_gl_ext.h"
174 #include "lg_cubemap.h"
175 #include "lg_error.h"
176 #include "lg_file_ops.h"
177 #include "lg_android_assets.h"
178 #include "lg_render.h"
179 #include "lg_terrain.h"
180 #include "lg_perlin_noise.h"
181 #include "lg_landscape.h"
184 LG_OK = LIBETM_LASTERRORCODE + 1,
187 LG_EXTRA_LIB_INIT_ERROR,
190 LG_CREATE_FILE_ERROR,
193 LG_READ_FROM_FILE_ERROR,
194 LG_WRITE_TO_FILE_ERROR,
195 LG_FILE_ACCESS_ERROR,
196 LG_READ_FROM_FILE_EOF,
210 LG_CONTINUE = LG_LASTERRORCODE + 1,
225 #define LG_WIN_WIDTH_MIN 800
226 #define LG_WIN_HEIGHT_MIN 400
228 #define LG_WIN_D_HEIGHT_HACK 2
231 #define LG_REQUESTED_RED_SIZE 5
232 #define LG_REQUESTED_GREEN_SIZE 6
233 #define LG_REQUESTED_BLUE_SIZE 5
234 #define LG_REQUESTED_ALPHA_SIZE 0
235 #define LG_REQUESTED_DEPTH_SIZE 16
236 #define LG_REQUESTED_STENCIL_SIZE 8
258 SDL_DisplayMode sdl_display_mode;
259 int sdl_convert_surf_format;
260 int sdl_create_rgb_surf_bitdepth;
261 int r_size, g_size, b_size, a_size;
262 uint32_t r_mask, g_mask, b_mask, a_mask;
272 SDL_GLContext gl_context;
279 int top_win_logical_w;
280 int top_win_logical_h;
283 float fullscreen_scale;
287 int max_combined_texture_image_units;
288 int max_texture_size;
289 int max_cubemap_texture_size;
293 GLuint lg_backgroud_tex_id;
302 char *org_name_android;
303 char *app_name_android;
311 zboolean enable_mouse;
315 zboolean oes_vao_extension;
316 void (*glGenVertexArraysOES)(GLsizei, GLuint *);
317 void (*glBindVertexArrayOES)(GLuint);
318 void (*glDeleteVertexArraysOES)(GLsizei,
const GLuint *);
319 void (*glIsVertexArrayOES)(GLuint);
320 zboolean oes_element_index_uint_extension;
321 zboolean ext_texcompr_s3tc_extension;
322 zboolean oes_texcompr_etc1_rgb8_extension;
323 zboolean khr_texcompr_astc_ldr_extension;
332 zboolean fonts_loaded;
335 #include "lg_env_instance.h"
337 #define GAME_FONT1 "DejaVuSans-Bold.ttf"
338 #define GAME_FONT2 "PAPYRUS.ttf"
339 #define GAME_FONT3 "intelone-mono-font-family-bold.ttf"
342 #define LEFT_ARROW_LIGHT_UTF8 "\u2190"
343 #define RIGHT_ARROW_LIGHT_UTF8 "\u2192"
344 #define UP_ARROW_LIGHT_UTF8 "\u2191"
345 #define DOWN_ARROW_LIGHT_UTF8 "\u2193"
348 #define UTF8_RW_ROUNDED_ARROW "\u279c"
350 int lg_init(
int,
int,
const char *,
const char *,
const char *,
const char *,
const char *,
const char *,
const char *);
int lg_load_fonts()
Definition: libgame.c:802
void lg_reset_viewport()
Definition: libgame.c:760
void lg_show_sys_info()
Definition: libgame.c:959
Definition: lg_audio.h:40
Definition: libgame.h:253
void lg_enable_mouse()
Definition: libgame.c:769
void lg_swap_fb()
Definition: libgame.c:927
Rec2Di lg_get_default_viewport()
Definition: libgame.c:742
Definition: lg_vertex.h:91
void lg_set_new_viewport(Rec2Di viewport)
Definition: libgame.c:752
int lg_init(int win_w, int win_h, const char *win_title, const char *app_name, const char *app_cmd, const char *org_name_android, const char *app_name_android, const char *assets_dir, const char *app_wr_dir)
Definition: libgame.c:51
void lg_show_extra_sys_info(SDL_Window *w)
Definition: libgame.c:1056
char * lg_get_sdl_win_flags(SDL_Window *w)
Definition: libgame.c:1131
zboolean sdl2_is_installed()
Definition: libgame.c:1115
void lg_quit(int exit_code)
Definition: libgame.c:840
Definition: lg_error.h:40
void lg_list_opengl_extensions()
Definition: libgame.c:785
void lg_disable_mouse()
Definition: libgame.c:777
void lg_show_lib_info()
Definition: libgame.c:935
Definition: lg_gr_func.h:47